WebSocket是 HTML5 开始提供的一种在单个 TCP 连接上进行全双工通讯的协议。
以前的推送技术使用 Ajax 轮询,浏览器需要不断地向服务器发送http请求来获取最新的数据,浪费很多的带宽等资源。
使用webSocket通讯,客户端和服务端只需要一次握手建立连接,就可以互相发送消息,进行数据传输,更实时地进行通讯。
一次握手建立WebSocket连接
浏览器先向服务器发送个url以ws://开头的http的GET请求,响应状态码101表示Switching Protocols切换协议,
服务器根据请求头中Upgrade:websocket把
客户端的请求切换到对应的协议,即websocket协议。
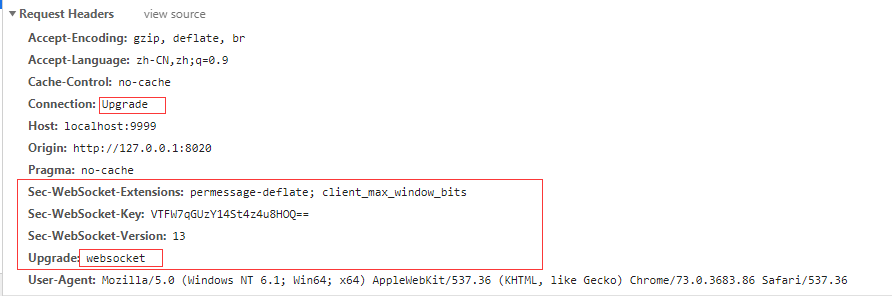
响应头消息中包含Upgrade:websocket,表示它切换到的协议,即websocket协议。
响应101,握手成功,http协议切换成websocket协议了,连接建立成功,浏览器和服务器可以随时主动发送消息给对方了,并且这个连接一直持续到客户端或服务器一方主动关闭连接。
为了加深理解,用websocket实现简单的在线聊天,先画个时序图,直观感受下流程。
网上有websocket在线测试工具,可以直接使用还是挺方便的,http://www.blue-zero.com/WebSocket/
自定义客户端代码:
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title>WebSocket</title> </head> <body> <input id="url" type="text" size="30" value="ws://localhost:9999/a/b" /> <button onclick="openWebSocket()">打开WebSocket连接</button> <br/><br/> <textarea id="text" type="text"></textarea> <button onclick="send()">发送消息</button> <hr/> <button onclick="closeWebSocket()">关闭WebSocket连接</button> <hr/> <div id="message"></div> </body> <script type="text/javascript"> var websocket = null; function openWebSocket() { var url = document.getElementById('url').value.trim(); //判断当前浏览器是否支持WebSocket if ('WebSocket' in window) { websocket = new WebSocket(url); } else { alert('当前浏览器 Not support websocket') } //连接发生错误的回调方法 websocket.onerror = function() { setMessageInnerHTML("WebSocket连接发生错误"); }; //连接成功建立的回调方法 websocket.onopen = function() { setMessageInnerHTML("WebSocket连接成功"); } //接收到消息的回调方法 websocket.onmessage = function(event) { setMessageInnerHTML(event.data); } //连接关闭的回调方法 websocket.onclose = function() { setMessageInnerHTML("WebSocket连接关闭"); } } //监听窗口关闭事件,当窗口关闭时,主动去关闭websocket连接,防止连接还没断开就关闭窗口,server端会抛异常。 window.onbeforeunload = function() { closeWebSocket(); } //将消息显示在网页上 function setMessageInnerHTML(innerHTML) { document.getElementById('message').innerHTML += innerHTML + '<br/>'; } //关闭WebSocket连接 function closeWebSocket() { websocket.close(); } //发送消息 function send() { var message = document.getElementById('text').value; websocket.send(message); } </script> </html>
服务端代码:
引入依赖,我使用的是springboot版本2.0.5.RELEASE,自动管理依赖版本
<dependency> <groupId>org.springframework.boot</groupId> <artifactId>spring-boot-starter-websocket</artifactId> <!-- <version>1.3.5.RELEASE</version> --> </dependency>
配置类WebSocketConfig,扫描并注册带有@ServerEndpoint注解的所有websocket服务端
package com.webSocket; import org.springframework.context.annotation.Bean; import org.springframework.context.annotation.Configuration; import org.springframework.web.socket.server.standard.ServerEndpointExporter; /** * 开启WebSocket支持 * @author Administrator */ @Configuration public class WebSocketConfig { /** * 扫描并注册带有@ServerEndpoint注解的所有服务端 * @return */ @Bean public ServerEndpointExporter serverEndpointExporter() { return new ServerEndpointExporter(); } }
WebSocketServer类,是websocket服务端,多例的,一次websocket连接对应一个实例
package com.webSocket; import java.io.IOException; import java.util.Collection; import java.util.concurrent.ConcurrentHashMap; import java.util.concurrent.atomic.AtomicInteger; import javax.websocket.*; import javax.websocket.server.PathParam; import javax.websocket.server.ServerEndpoint; import org.springframework.stereotype.Component; /** * websocket服务端,多例的,一次websocket连接对应一个实例 * @ServerEndpoint 注解的值为URI,映射客户端输入的URL来连接到WebSocket服务器端 */ @Component @ServerEndpoint("/{name}/{toName}") public class WebSocketServer { /** 用来记录当前在线连接数。设计成线程安全的。*/ private static AtomicInteger onlineCount = new AtomicInteger(0); /** 用于保存uri对应的连接服务,{uri:WebSocketServer},设计成线程安全的 */ private static ConcurrentHashMap<String, WebSocketServer> webSocketServerMAP = new ConcurrentHashMap<>(); private Session session;// 与某个客户端的连接会话,需要通过它来给客户端发送数据 private String name; //客户端消息发送者 private String toName; //客户端消息接受者 private String uri; //连接的uri /** * 连接建立成功时触发,绑定参数 * @param session * 可选的参数。session为与某个客户端的连接会话,需要通过它来给客户端发送数据 * @param name * @param toName * @throws IOException */ @OnOpen public void onOpen(Session session, @PathParam("name") String name, @PathParam("toName") String toName) throws IOException { this.session = session; this.name = name; this.toName = toName; this.uri = session.getRequestURI().toString(); WebSocketServer webSocketServer = webSocketServerMAP.get(uri); if(webSocketServer != null){ //同样业务的连接已经在线,则把原来的挤下线。 webSocketServer.session.getBasicRemote().sendText(uri + "重复连接被挤下线了"); webSocketServer.session.close();//关闭连接,触发关闭连接方法onClose() } webSocketServerMAP.put(uri, this);//保存uri对应的连接服务 addOnlineCount(); // 在线数加1 sendMessage(webSocketServerMAP +"当前在线连接数:" + getOnlineCount()); System.out.println(this + "有新连接加入!当前在线连接数:" + getOnlineCount()); } /** * 连接关闭时触发,注意不能向客户端发送消息了 * @throws IOException */ @OnClose public void onClose() throws IOException { webSocketServerMAP.remove(uri);//删除uri对应的连接服务 reduceOnlineCount(); // 在线数减1 System.out.println("有一连接关闭!当前在线连接数" + getOnlineCount()); } /** * 收到客户端消息后触发,分别向2个客户端(消息发送者和消息接收者)推送消息 * * @param message * 客户端发送过来的消息 * @param session * 可选的参数 * @throws IOException */ @OnMessage public void onMessage(String message) throws IOException { //服务器向消息发送者客户端发送消息 this.session.getBasicRemote().sendText("发送给" + toName + "的消息:" + message); //获取消息接收者的客户端连接 StringBuilder receiverUri = new StringBuilder("/"); receiverUri.append(toName) .append("/") .append(name); WebSocketServer webSocketServer = webSocketServerMAP.get(receiverUri.toString()); if(webSocketServer != null){ webSocketServer.session.getBasicRemote().sendText("来自" +name + "的消息:" + message); } /*// 群发消息 Collection<WebSocketServer> collection = webSocketServerMAP.values(); for (WebSocketServer item : collection) { try { item.sendMessage("收到群发消息:" + message); } catch (IOException e) { e.printStackTrace(); continue; } }*/ } /** * 通信发生错误时触发 * * @param session * @param error */ @OnError public void onError(Session session, Throwable error) { System.out.println("发生错误"); error.printStackTrace(); } /** * 向客户端发送消息 * @param message * @throws IOException */ public void sendMessage(String message) throws IOException { this.session.getBasicRemote().sendText(message); } /** * 获取在线连接数 * @return */ public static int getOnlineCount() { return onlineCount.get(); } /** * 原子性操作,在线连接数加一 */ public static void addOnlineCount() { onlineCount.getAndIncrement(); } /** * 原子性操作,在线连接数减一 */ public static void reduceOnlineCount() { onlineCount.getAndDecrement(); } }
启动项目,我这里的端口号为9999,websocket服务端就可以使用了。然后打开两个客户端,打开连接ws://localhost:9999/a/b和ws://localhost:9999/b/a,就可以进行通讯了
1.使用客户端a向b发送消息:
客户端b收到消息:
2.使用客户端b向a发送消息:
客户端a收到消息:
这样使用websocket简单在线聊天实践了下,还有很多细节没有实现,主要是加深下websocket的理解,以后再慢慢完善。
相比http,减少了请求次数,不需要客户端多次请求,服务器处理业务完毕后主动向客户端推送消息。
来源:https://www.cnblogs.com/liuyong1993/p/10718961.html