一、环境
- Idea 2020.1
- JDK 1.8
- maven
二、目的
spring boot整合cache
三、步骤
3.1、点击File -> New Project -> Spring Initializer,点击next
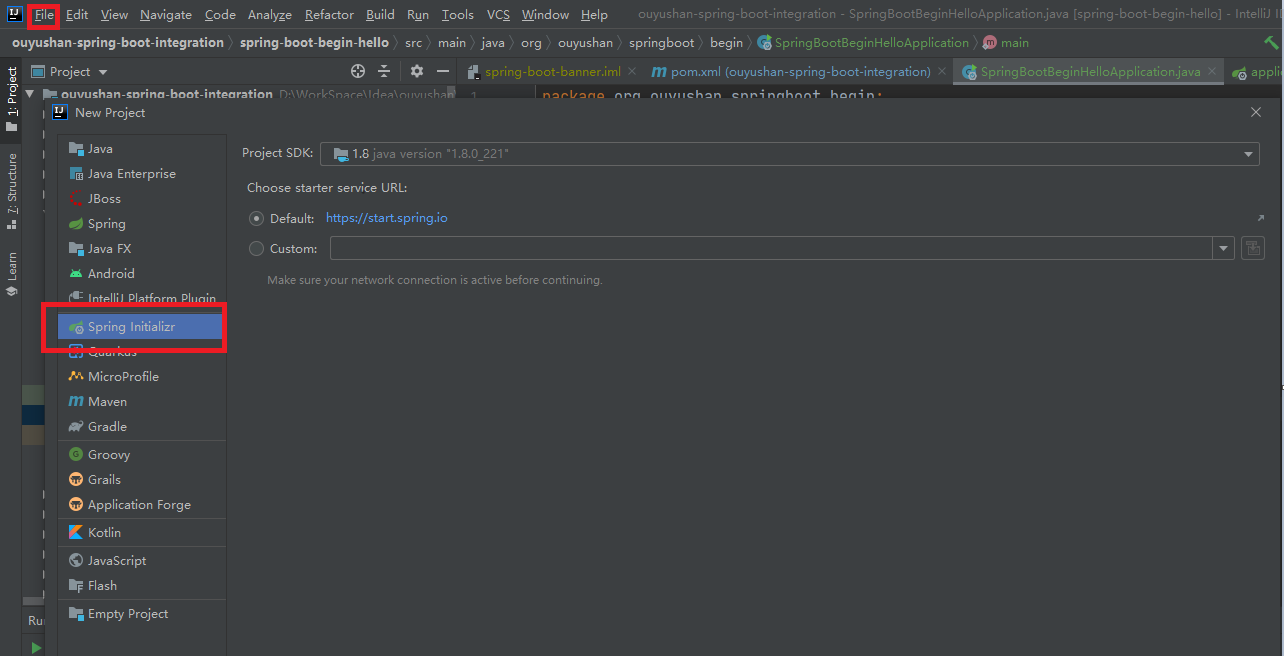
3.2、在对应地方修改自己的项目信息
3.3、选择Web依赖,选中Spring Web、Spring Boot Actuator。可以选择Spring Boot版本,本次默认为2.2.7,点击Next
3.4、项目结构
四、添加文件
pom.xml文件
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.2.7.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>org.ouyushan</groupId>
<artifactId>spring-boot-data-cache</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>spring-boot-data-cache</name>
<description>Cache project for Spring Boot</description>
<properties>
<java.version>1.8</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Compile -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-cache</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
<exclusions>
<exclusion>
<groupId>org.junit.vintage</groupId>
<artifactId>junit-vintage-engine</artifactId>
</exclusion>
</exclusions>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
<!-- 各版本缓存依赖 -->
<profiles>
<profile>
<id>caffeine</id>
<dependencies>
<dependency>
<groupId>com.github.ben-manes.caffeine</groupId>
<artifactId>caffeine</artifactId>
</dependency>
</dependencies>
</profile>
<profile>
<id>couchbase</id>
<dependencies>
<dependency>
<groupId>com.couchbase.client</groupId>
<artifactId>java-client</artifactId>
</dependency>
<dependency>
<groupId>com.couchbase.client</groupId>
<artifactId>couchbase-spring-cache</artifactId>
</dependency>
</dependencies>
</profile>
<profile>
<id>ehcache2</id>
<dependencies>
<dependency>
<groupId>net.sf.ehcache</groupId>
<artifactId>ehcache</artifactId>
</dependency>
</dependencies>
</profile>
<profile>
<id>ehcache</id>
<dependencies>
<dependency>
<groupId>javax.cache</groupId>
<artifactId>cache-api</artifactId>
</dependency>
<dependency>
<groupId>org.ehcache</groupId>
<artifactId>ehcache</artifactId>
</dependency>
</dependencies>
</profile>
<profile>
<id>hazelcast</id>
<dependencies>
<dependency>
<groupId>com.hazelcast</groupId>
<artifactId>hazelcast</artifactId>
</dependency>
<dependency>
<groupId>com.hazelcast</groupId>
<artifactId>hazelcast-spring</artifactId>
</dependency>
</dependencies>
</profile>
<profile>
<id>infinispan</id>
<dependencies>
<dependency>
<groupId>org.infinispan</groupId>
<artifactId>infinispan-spring4-embedded</artifactId>
</dependency>
<dependency>
<groupId>org.infinispan</groupId>
<artifactId>infinispan-jcache</artifactId>
</dependency>
</dependencies>
</profile>
<profile>
<id>redis</id>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
</dependencies>
</profile>
</profiles>
</project>
Country.java文件
package org.ouyushan.springboot.data.cache.entity;
import java.io.Serializable;
/**
* @Description:
* @Author: ouyushan
* @Email: ouyushan@hotmail.com
* @Date: 2020/5/8 15:25
*/
public class Country implements Serializable {
private final String code;
public Country(String code) {
this.code = code;
}
public String getCode() {
return this.code;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
Country country = (Country) o;
return this.code.equals(country.code);
}
@Override
public int hashCode() {
return this.code.hashCode();
}
}
CountryRepository.java
package org.ouyushan.springboot.data.cache.dao;
import org.ouyushan.springboot.data.cache.entity.Country;
import org.springframework.cache.annotation.CacheConfig;
import org.springframework.cache.annotation.Cacheable;
import org.springframework.stereotype.Component;
/**
* @Description: 通过注解@CacheConfig配置缓存名称
* @Author: ouyushan
* @Email: ouyushan@hotmail.com
* @Date: 2020/5/8 15:26
*/
@Component
// 配置缓存名称
@CacheConfig(cacheNames = "countries")
public class CountryRepository {
@Cacheable
public Country findByCode(String code) {
System.out.println("---> Loading country with code '" + code + "'");
return new Country(code);
}
}
CacheManagerCheck.java
package org.ouyushan.springboot.data.cache.check;
/**
* @Description: 获取缓存实现方式
* @Author: ouyushan
* @Email: ouyushan@hotmail.com
* @Date: 2020/5/8 15:24
*/
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.springframework.boot.CommandLineRunner;
import org.springframework.cache.CacheManager;
import org.springframework.stereotype.Component;
@Component
public class CacheManagerCheck implements CommandLineRunner {
private static final Log logger = LogFactory.getLog(CacheManagerCheck.class);
private final CacheManager cacheManager;
public CacheManagerCheck(CacheManager cacheManager) {
this.cacheManager = cacheManager;
}
@Override
public void run(String... strings) throws Exception {
logger.info("\n\n" + "=========================================================\n" + "Using cache manager: "
+ this.cacheManager.getClass().getName() + "\n"
+ "=========================================================\n\n");
}
}
CacheJob.java
package org.ouyushan.springboot.data.cache.schedule;
import org.ouyushan.springboot.data.cache.dao.CountryRepository;
import org.springframework.context.annotation.Profile;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
import java.util.Arrays;
import java.util.List;
import java.util.Random;
/**
* @Description: 验证缓存的定时任务
* @Author: ouyushan
* @Email: ouyushan@hotmail.com
* @Date: 2020/5/8 15:33
*/
@Component
@Profile("app")
public class CacheJob {
private static final List<String> SAMPLE_COUNTRY_CODES = Arrays.asList("AF", "AX", "AL", "DZ", "AS", "AD", "AO",
"AI", "AQ", "AG", "AR", "AM", "AW", "AU", "AT", "AZ", "BS", "BH", "BD", "BB", "BY", "BE", "BZ", "BJ", "BM",
"BT", "BO", "BQ", "BA", "BW", "BV", "BR", "IO", "BN", "BG", "BF", "BI", "KH", "CM", "CA", "CV", "KY", "CF",
"TD", "CL", "CN", "CX", "CC", "CO", "KM", "CG", "CD", "CK", "CR", "CI", "HR", "CU", "CW", "CY", "CZ", "DK",
"DJ", "DM", "DO", "EC", "EG", "SV", "GQ", "ER", "EE", "ET", "FK", "FO", "FJ", "FI", "FR", "GF", "PF", "TF",
"GA", "GM", "GE", "DE", "GH", "GI", "GR", "GL", "GD", "GP", "GU", "GT", "GG", "GN", "GW", "GY", "HT", "HM",
"VA", "HN", "HK", "HU", "IS", "IN", "ID", "IR", "IQ", "IE", "IM", "IL", "IT", "JM", "JP", "JE", "JO", "KZ",
"KE", "KI", "KP", "KR", "KW", "KG", "LA", "LV", "LB", "LS", "LR", "LY", "LI", "LT", "LU", "MO", "MK", "MG",
"MW", "MY", "MV", "ML", "MT", "MH", "MQ", "MR", "MU", "YT", "MX", "FM", "MD", "MC", "MN", "ME", "MS", "MA",
"MZ", "MM", "NA", "NR", "NP", "NL", "NC", "NZ", "NI", "NE", "NG", "NU", "NF", "MP", "NO", "OM", "PK", "PW",
"PS", "PA", "PG", "PY", "PE", "PH", "PN", "PL", "PT", "PR", "QA", "RE", "RO", "RU", "RW", "BL", "SH", "KN",
"LC", "MF", "PM", "VC", "WS", "SM", "ST", "SA", "SN", "RS", "SC", "SL", "SG", "SX", "SK", "SI", "SB", "SO",
"ZA", "GS", "SS", "ES", "LK", "SD", "SR", "SJ", "SZ", "SE", "CH", "SY", "TW", "TJ", "TZ", "TH", "TL", "TG",
"TK", "TO", "TT", "TN", "TR", "TM", "TC", "TV", "UG", "UA", "AE", "GB", "US", "UM", "UY", "UZ", "VU", "VE",
"VN", "VG", "VI", "WF", "EH", "YE", "ZM", "ZW");
private final CountryRepository countryService;
private final Random random;
CacheJob(CountryRepository countryService) {
this.countryService = countryService;
this.random = new Random();
}
@Scheduled(fixedDelay = 500)
public void retrieveCountry() {
String randomCode = SAMPLE_COUNTRY_CODES.get(this.random.nextInt(SAMPLE_COUNTRY_CODES.size()));
System.out.println("Looking for country with code '" + randomCode + "'");
this.countryService.findByCode(randomCode);
}
}
SpringBootDataCacheApplication.java
package org.ouyushan.springboot.data.cache;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.builder.SpringApplicationBuilder;
import org.springframework.cache.annotation.EnableCaching;
import org.springframework.scheduling.annotation.EnableScheduling;
@EnableCaching
@EnableScheduling
@SpringBootApplication
public class SpringBootDataCacheApplication {
public static void main(String[] args) {
new SpringApplicationBuilder().sources(SpringBootDataCacheApplication.class).profiles("app").run(args);
}
}
四、测试
运行以下测试用例
SpringBootDataCacheApplicationTests .java
package org.ouyushan.springboot.data.cache;
import org.junit.jupiter.api.Test;
import org.ouyushan.springboot.data.cache.dao.CountryRepository;
import org.ouyushan.springboot.data.cache.entity.Country;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.cache.Cache;
import org.springframework.cache.CacheManager;
import static org.assertj.core.api.Assertions.assertThat;
@SpringBootTest
class SpringBootDataCacheApplicationTests {
@Autowired
private CacheManager cacheManager;
@Autowired
private CountryRepository countryRepository;
@Test
public void validateCache() {
Cache countries = this.cacheManager.getCache("countries");
assertThat(countries).isNotNull();
countries.clear(); // Simple test assuming the cache is empty
assertThat(countries.get("BE")).isNull();
Country be = this.countryRepository.findByCode("BE");
assertThat((Country) countries.get("BE").get()).isEqualTo(be);
}
}
或者更改application中的配置以及启动中的profile测试其他
来源:oschina
链接:https://my.oschina.net/ouyushan/blog/4303867